R Generate Random 0 and 1
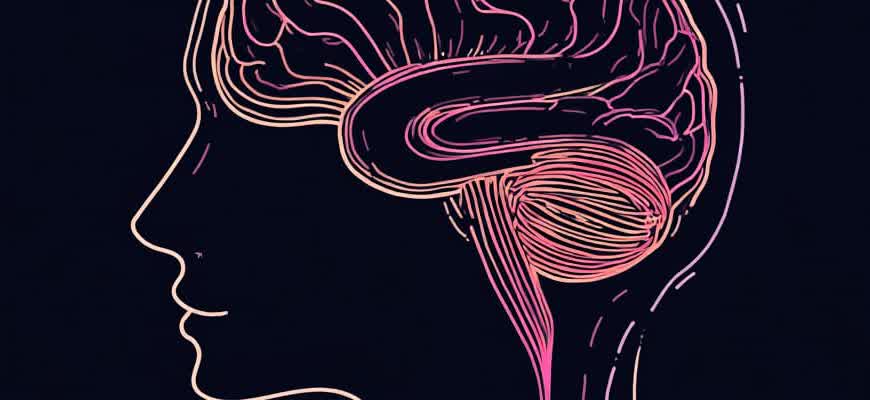
In R, one of the most common tasks is generating random binary values. These values, either 0 or 1, can be used in a variety of applications, from simulations to statistical modeling. Below is a guide on how to generate random binary numbers in R using different functions and methods.
1. Using the sample() function
The sample()
function in R is versatile and allows you to generate random binary values. It randomly selects values from a specified set of numbers, in this case, 0
and 1
.
- Syntax:
sample(c(0, 1), size = n, replace = TRUE)
- n is the number of values to generate.
- replace = TRUE allows for repeated values.
Example:
sample(c(0, 1), size = 10, replace = TRUE)
This generates a random sequence of 10 binary numbers.
2. Using the rbinom() function
Another powerful function in R is rbinom()
, which generates random binary numbers based on a binomial distribution.
- Syntax:
rbinom(n, size = 1, prob = 0.5)
- n is the number of random values to generate.
- size = 1 indicates a single trial for each random value.
- prob is the probability of generating a 1 (default is 0.5).
Example:
rbinom(10, size = 1, prob = 0.5)
This will generate 10 random binary values with a 50% chance of being 1.
Function | Purpose |
---|---|
sample() |
Generates random binary values from a given set of numbers. |
rbinom() |
Generates random binary values based on a binomial distribution. |
Setting Up the R Environment for Random 0 and 1 Generation
To generate random sequences of 0s and 1s in R, the first step is to set up the appropriate environment. This ensures that the required packages and functions are readily available for generating binary data. By default, R provides various tools to create random numbers, and these can be used to simulate binary outcomes. Understanding how to configure these tools will make the process seamless and efficient.
In this guide, we will cover the essential setup steps and the functions most commonly used for generating random binary sequences in R. This includes loading the necessary libraries, selecting the right functions, and configuring parameters for random number generation.
Step 1: Installing and Loading Required Packages
The R environment comes with built-in functions that can handle random number generation, but some advanced tools might require additional packages. Start by ensuring that your R installation is up to date and install any necessary libraries using the following approach:
- Open your R console or RStudio.
- Install the necessary packages (if needed) using the install.packages() function.
- Load the required libraries using the library() function.
Note: The base package already includes functions like sample() and runif() that are commonly used for generating random binary data.
Step 2: Generating Random Binary Sequences
Once your environment is set up, generating random binary values is straightforward. The two most commonly used functions are sample() and runif(), which can be customized for different purposes:
- sample() is ideal for generating random samples with specific probabilities for 0 and 1.
- runif() can be used to generate uniformly distributed random numbers, which can then be converted to 0s and 1s based on a threshold value.
Function | Description | Example |
---|---|---|
sample() | Generates a random sample of 0s and 1s. | sample(c(0, 1), size = 10, replace = TRUE) |
runif() | Generates random numbers, which can be thresholded to produce binary values. | ifelse(runif(10) > 0.5, 1, 0) |
Troubleshooting Common Issues When Generating Random 0s and 1s in R
Generating random binary values in R is a simple task, but users can sometimes run into issues that can affect the accuracy or performance of their code. The process typically involves using functions like sample() or rbinom(), but common mistakes or misunderstandings can cause unexpected results. Understanding and resolving these issues is key to ensuring correct random number generation.
Here are some frequent challenges and how to fix them when generating random binary numbers in R.
1. Incorrect Sample Size
One of the most common issues is specifying an incorrect sample size. If the size is set incorrectly or not explicitly defined, the function may not behave as expected.
Remember: Always double-check the size argument to ensure you are generating the correct number of random values.
- Check if the argument size in functions like sample() matches your intended output.
- Ensure the range of values (0 and 1) is set correctly.
2. Non-Uniform Distribution
Another issue could be the generation of biased outcomes where one value (0 or 1) appears more frequently than the other. This could be caused by incorrect probability settings.
If you need a uniform distribution, make sure the probability is set to 0.5 for both 0 and 1.
- In sample(), ensure that the prob argument is not skewed.
- For a fair random distribution, the probabilities for 0 and 1 should both be 0.5.
3. Incorrect Use of Random Binomial Generation
Using rbinom() without understanding the parameters can lead to problems, especially when the number of trials or probability is misconfigured.
Parameter | Meaning |
---|---|
n | Number of random samples to generate. |
size | Number of trials in each experiment (set to 1 for binary results). |
prob | Probability of success (set to 0.5 for uniform distribution). |
By ensuring proper understanding and application of parameters, you can avoid many common issues related to generating random binary numbers in R.
Optimizing R Code for Faster Random Number Generation
Efficient random number generation is crucial for simulations and data analysis in R. Whether generating binary values, continuous distributions, or large datasets, optimizing the speed of random number generation can significantly improve performance, especially when dealing with large datasets or repeated simulations. The R environment provides several methods to generate random values, each with varying performance characteristics based on use cases.
To achieve faster execution times, it's essential to understand how R handles random number generation and how specific techniques and packages can enhance performance. By leveraging optimized libraries or adjusting algorithm parameters, the process of generating random sequences becomes more efficient and resource-friendly.
Techniques for Improving Random Number Generation Performance
- Use of
RNGkind()
to control RNG type: The default random number generator in R may not always be the fastest. You can use theRNGkind()
function to specify a different generator, such as theMersenne-Twister
orSuper-Duper
, which may offer better performance in certain cases. - Vectorization of operations: Instead of generating random numbers in a loop, use vectorized operations which are typically faster in R. Functions like
sample()
orrunif()
work well when handling large data in one go. - Parallel processing: For large-scale simulations, parallelization using packages like
parallel
orfuture
can distribute random number generation tasks across multiple cores, significantly speeding up the process.
Best Practices for Random Number Generation
- Choose the right generator for the task: Consider the trade-offs between different random number generators in terms of speed and quality.
- Avoid repetitive reseeding: Continuously reseeding the random number generator can be inefficient. Instead, generate random numbers in bulk with fewer calls to seed the generator.
- Use high-performance packages: Consider packages like
Rcpp
orrandtoolbox
for generating random numbers efficiently in C++ or specialized algorithms.
Performance Comparison
Method | Performance (time) | Use Case |
---|---|---|
Base R (sample) | Medium | General purpose random sampling |
Rcpp-based generation | Fast | High-performance applications |
Parallel processing (future) | Very Fast | Large-scale simulations |
Optimizing random number generation is not only about speed but also about choosing the right balance between quality and performance for your specific application.
Comparing Random Number Generation Methods in R
R provides several methods for generating random numbers, each with different characteristics and use cases. Understanding how these methods work and when to use them is crucial for ensuring the integrity of simulations, statistical analysis, and randomized algorithms. In this comparison, we will explore the most common random number generation techniques and highlight their strengths and weaknesses.
The most widely used approach is to generate random numbers from a uniform distribution, but R also supports normal, binomial, and other distributions. These different methods may have varying levels of performance, quality, and reproducibility. Below is a detailed comparison of the primary techniques for generating random numbers in R.
Uniform Distribution Method: runif()
The function runif() is commonly used to generate random numbers uniformly distributed between 0 and 1. This method is fast and efficient, making it a popular choice for simulations that require random sampling from this interval.
- Function:
runif(n, min = 0, max = 1)
- Returns: A vector of n random numbers between min and max
- Use case: Simulations, random sampling, and Monte Carlo methods
Normal Distribution Method: rnorm()
For generating random numbers that follow a normal (Gaussian) distribution, rnorm() is the preferred function. It allows users to specify the mean and standard deviation of the distribution.
- Function:
rnorm(n, mean = 0, sd = 1)
- Returns: A vector of n random numbers from a normal distribution
- Use case: Statistical analysis, hypothesis testing, and simulations requiring normality
Random Binary Numbers: sample()
To generate random binary numbers (0s and 1s), the sample() function is often used. This function provides more control over the probabilities of each outcome and can generate binary sequences of any length.
- Function:
sample(c(0, 1), size = n, replace = TRUE)
- Returns: A vector of n random binary numbers
- Use case: Randomized algorithms, simulations of binary processes, and binary decision models
Comparison Table
Method | Function | Distribution | Use Case |
---|---|---|---|
Uniform Distribution | runif() | Uniform (0, 1) | General-purpose simulations, random sampling |
Normal Distribution | rnorm() | Normal | Statistical analysis, hypothesis testing |
Binary Numbers | sample() | Binomial (0, 1) | Randomized algorithms, binary models |
Important: When performing simulations or statistical modeling, it's crucial to understand the characteristics of the random number generator in use, as the quality of randomness can impact the validity of your results.
Real-World Applications of Binary Random Generation in Data Science
In data science, the generation of random binary values (0s and 1s) plays a significant role in numerous real-world applications. These binary sequences are often employed to simulate various processes, test algorithms, and model uncertainties. Whether used for random sampling, data augmentation, or probabilistic simulations, generating random 0s and 1s is a fundamental operation that has far-reaching implications in various domains, including machine learning, statistics, and operations research.
One of the key benefits of using binary random values is their ability to simplify complex problems. By leveraging random binary sequences, data scientists can perform simulations of various systems, generate stochastic models, and estimate probabilities for uncertain events. The simplicity of binary values also makes them ideal for computational efficiency, which is crucial when handling large datasets and running performance-intensive tasks.
Applications in Real-World Data Science
- Random Sampling - Random binary sequences are frequently used in random sampling techniques such as Monte Carlo simulations or bootstrapping methods. These methods rely on generating random binary numbers to select data points or perform sampling with replacement.
- Feature Selection - In machine learning, random binary vectors are used to select features from a dataset for further analysis. Feature selection algorithms often use binary masks to represent whether a particular feature should be included in a model.
- Simulating Random Events - For probabilistic models, generating random binary outcomes simulates real-world scenarios like coin flips, stock price movements, or customer behavior. This is essential in predictive analytics and risk assessment.
Examples of Random Binary Generation in Data Science
- Creating Synthetic Datasets - In situations where collecting real data is challenging, random binary sequences can be used to generate synthetic datasets. These datasets can then be used to train models or test algorithms without relying on actual data.
- Evaluating Machine Learning Models - During model evaluation, random binary outcomes are used in cross-validation techniques, where the data is randomly divided into training and testing sets.
- Algorithm Benchmarking - Random 0s and 1s are useful in testing the performance of algorithms, as they can simulate worst-case scenarios and help assess the efficiency of various data processing methods.
Important: Random binary sequences provide a robust way to simulate uncertainty, test models, and evaluate algorithms, playing a vital role in enhancing the flexibility and accuracy of data-driven systems.
Table of Binary Random Generation Use Cases
Use Case | Application | Tools/Methods |
---|---|---|
Monte Carlo Simulations | Simulating random processes for predictive analysis | Random Binary Generation, Statistical Sampling |
Feature Selection | Selecting relevant features in machine learning models | Random Binary Vectors, Genetic Algorithms |
Data Augmentation | Creating additional training data by generating random binary patterns | Random Sampling, Data Manipulation |
Automating Binary Data Generation for Large Datasets in R
Generating binary data automatically is essential when working with large datasets in R. This technique is particularly useful when testing algorithms, simulating experiments, or performing data validation. By leveraging R’s built-in functions, users can create random sequences of 0s and 1s efficiently, which can be scaled up for large datasets.
Automating this process ensures reproducibility and saves time when generating datasets for testing machine learning models or other data analysis tasks. It is crucial to set parameters for controlling the data distribution, ensuring it matches the desired characteristics for each specific use case.
Methods for Binary Data Generation
- Using the sample function: This method allows the generation of random binary sequences with specified probabilities.
- Utilizing the rbinom function: A more specialized function for generating binary data from a binomial distribution.
- Creating custom binary sequences: Users can define custom distributions for generating binary data according to specific requirements.
Step-by-Step Guide to Generate Binary Data
- Choose the generation method: Decide whether to use the sample() or rbinom() function based on the required distribution.
- Define dataset size: Specify the number of binary elements (0s and 1s) required for the dataset.
- Set probability parameters: If needed, adjust the probability distribution for 0s and 1s to simulate realistic data distributions.
- Run the function: Execute the chosen method to generate the binary data.
- Verify the output: Inspect the generated data for accuracy and distribution consistency.
It is important to remember that random binary data generation is not just about creating sequences; the pattern and distribution should align with the specific use case of the analysis.
Example of a Random Binary Dataset Generation
Method | Code Example |
---|---|
Using sample() | sample(0:1, size = 1000, replace = TRUE) |
Using rbinom() | rbinom(1000, 1, prob = 0.5) |