How to Create an Ai From Scratch
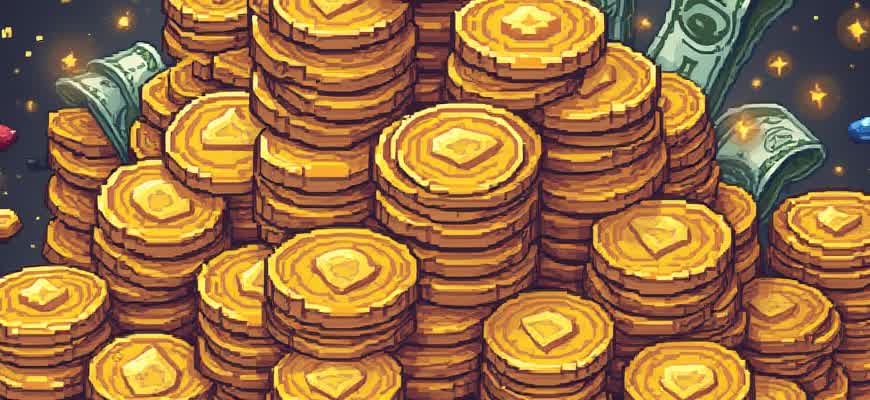
Creating an AI from scratch is a multi-step process that involves a combination of software engineering, mathematical modeling, and data processing. To begin, one must first understand the fundamentals of AI systems and the types of problems they aim to solve. Below is a breakdown of the core components that need to be considered:
- Problem Identification - Define the specific problem the AI will address.
- Data Collection - Gather and preprocess relevant datasets for training purposes.
- Model Selection - Choose an appropriate machine learning model based on the problem.
- Algorithm Development - Develop algorithms that will allow the AI to learn from data.
After these initial steps, you can move on to model training and optimization. However, some key principles should guide your process:
Success in AI development largely depends on the quality of the data and the model's ability to generalize, meaning it can apply learned patterns to new, unseen data.
Here’s a brief outline of the typical process:
- Data Preparation - Clean and format the data to ensure it’s suitable for training.
- Model Training - Train the selected model using the prepared dataset.
- Evaluation - Test the model's performance and make adjustments as needed.
- Deployment - Implement the AI into a real-world environment where it can be used.
Throughout this journey, continual evaluation and refinement are crucial for building a successful AI system. Below is a table summarizing some of the main methods and tools used at each stage:
Stage | Key Methods/Tools |
---|---|
Data Collection | Web Scraping, APIs, Data Augmentation |
Model Selection | Linear Regression, Neural Networks, Decision Trees |
Training | Gradient Descent, Backpropagation, Cross-Validation |
Evaluation | Accuracy, Precision, Recall, F1-Score |
Building an AI from the Ground Up
Creating an AI from scratch involves multiple stages, from defining the problem to deploying the system. The process begins with selecting an appropriate algorithm and designing the structure of the AI system. Machine learning models, such as supervised or unsupervised learning, are commonly used to train the system based on large datasets. A well-designed architecture is crucial to ensure the AI can generalize well to new data and perform efficiently in real-world situations.
Once the architecture is set, the next step is to focus on data collection, cleaning, and preprocessing. The quality of the data is vital for training a robust AI model. Afterward, the system is trained, evaluated, and iterated to improve its performance. Here are the main steps involved:
Steps to Create an AI System
- Problem Definition: Clearly define the problem you want the AI to solve.
- Data Collection: Gather relevant and high-quality data for training the model.
- Data Preprocessing: Clean and normalize the data to make it suitable for training.
- Algorithm Selection: Choose the most appropriate machine learning algorithm based on the problem type.
- Model Training: Train the model using the prepared data.
- Model Evaluation: Assess the model's performance and adjust parameters to improve accuracy.
- Deployment: Deploy the AI system in a real-world environment for use.
"The foundation of AI creation lies in data. Without quality data, even the best algorithms cannot perform well."
Here’s an example of how different algorithms might perform based on the type of problem:
Problem Type | Recommended Algorithm | Use Case Example |
---|---|---|
Classification | Random Forest, Support Vector Machine | Email spam detection, Image recognition |
Regression | Linear Regression, Decision Trees | Predicting house prices, Stock forecasting |
Clustering | K-Means, DBSCAN | Customer segmentation, Anomaly detection |
Understanding the Fundamentals of AI Development
Artificial Intelligence (AI) development requires a solid understanding of core concepts, algorithms, and techniques. Building an AI system from scratch involves selecting the right tools and frameworks while also grasping how different AI components interact. Developers must familiarize themselves with mathematical foundations, programming languages, and problem-solving strategies to create effective AI models.
The key to successful AI development lies in mastering three critical areas: data processing, model training, and evaluation. AI systems rely heavily on data, which must be preprocessed and transformed into a format suitable for model input. After training, models must be rigorously tested to ensure they perform as expected in real-world scenarios.
Key Areas in AI Development
- Data Acquisition and Preprocessing: Collecting relevant data and cleaning it for model input.
- Algorithm Selection: Choosing the right machine learning algorithm (e.g., supervised, unsupervised, reinforcement learning).
- Model Training: Using data to train the algorithm and tune the model's parameters.
- Testing and Evaluation: Validating model performance using metrics like accuracy, precision, and recall.
Core Algorithms in AI
- Decision Trees: Used for classification and regression tasks.
- Neural Networks: Powerful models for tasks like image and speech recognition.
- Support Vector Machines (SVM): Applied for classification tasks with high-dimensional data.
- Clustering Algorithms: For grouping data based on similarities.
Important: Mastery of algorithms is essential for AI development. Without a deep understanding of how different algorithms work, it's difficult to choose the best solution for a given problem.
AI Tools and Frameworks
Tool | Purpose |
---|---|
TensorFlow | Open-source framework for machine learning and deep learning. |
PyTorch | Another popular framework for deep learning research and production. |
Scikit-learn | Library for machine learning in Python, focused on simplicity and efficiency. |
Keras | High-level neural networks API built on top of TensorFlow. |
Choosing the Right Programming Language for AI Projects
When starting an AI project, selecting the right programming language is critical for the efficiency, scalability, and maintainability of the solution. Different languages offer various libraries, frameworks, and tools that make AI development easier and more efficient. The choice largely depends on the specific requirements of the project, such as the type of AI model, the complexity, and the resources available.
Each programming language has its strengths and weaknesses, which influence how it performs in AI development. Understanding the differences and how they align with the project goals can save a lot of time and effort in the long run.
Popular Languages for AI Development
- Python - Known for its simplicity and a wide range of AI libraries like TensorFlow, PyTorch, and scikit-learn. Ideal for machine learning and deep learning tasks.
- R - Often used in statistics and data analysis, it is suitable for data-heavy AI projects, including those in the healthcare and financial sectors.
- Java - Offers good performance and scalability, used in AI systems requiring robustness, such as large-scale applications.
- C++ - Preferred for AI in performance-critical applications, including computer vision and robotics.
- JavaScript - Gaining traction for AI in web applications, especially for real-time data processing and front-end implementations.
Key Considerations
- Project Requirements: Consider what the AI project needs. If it's deep learning-focused, Python is usually the go-to. For systems requiring low latency, C++ may be more appropriate.
- Community and Libraries: A strong community and access to mature libraries significantly improve development speed. Python excels in this area with its vast AI ecosystem.
- Learning Curve: Choose a language that aligns with the team's skillset. Python, for instance, has a gentle learning curve, while Java or C++ might require more expertise.
Language Comparison
Language | Use Case | Advantages | Disadvantages |
---|---|---|---|
Python | Machine Learning, Deep Learning, Data Science | Wide library support, simple syntax, large community | Not as fast as C++ or Java |
R | Data Analysis, Statistical Modelling | Strong statistical support, specialized libraries | Less general-purpose than Python |
Java | Large-scale AI systems, AI in production | Scalable, portable, strong performance | Verbose syntax, slower development speed |
C++ | Performance-critical AI systems (e.g., robotics) | High performance, control over memory management | Complex syntax, steep learning curve |
JavaScript | AI in web development, real-time processing | Integration with web technologies, growing support for AI | Limited AI libraries compared to Python |
Tip: Start with Python if you are new to AI development. Its simplicity and large ecosystem make it a great entry point before moving to more complex languages if needed.
Setting Up Your Development Environment for AI
Before diving into the development of an AI system, it's crucial to set up a proper development environment. This will provide the necessary tools, libraries, and configurations to build, train, and deploy models efficiently. The environment setup includes selecting the right programming languages, frameworks, and ensuring compatibility across all components.
In this section, we will walk through the essential steps to configure your system for AI development, covering software installation, setting up dependencies, and optimizing your workspace for smooth workflow.
1. Choosing the Right Programming Language
The choice of programming language plays a significant role in AI development. Most AI projects use Python due to its extensive library support and ease of use. Other languages such as R, Java, and Julia can also be used, depending on specific requirements.
- Python: Most commonly used due to libraries like TensorFlow, PyTorch, and Scikit-learn.
- R: Preferred in statistics-heavy tasks, particularly in data science.
- Java: Useful for large-scale production systems due to its speed and scalability.
- Julia: Gaining traction for high-performance computing tasks.
2. Installing Essential Libraries
Once the programming language is chosen, the next step is to install AI libraries. Libraries like TensorFlow and PyTorch are commonly used for deep learning, while others like Scikit-learn and Pandas serve general-purpose data manipulation and machine learning tasks.
- TensorFlow: Used for deep learning and neural networks.
- PyTorch: Another deep learning library known for flexibility and speed.
- Scikit-learn: Great for classical machine learning algorithms.
- Pandas: For data manipulation and preprocessing.
3. Setting Up the Development Environment
For efficient AI development, it's important to set up a dedicated environment that keeps dependencies isolated. This can be done using tools like virtualenv or conda.
Tool | Description |
---|---|
virtualenv | Creates isolated Python environments for each project. |
conda | Manages environments and dependencies for various programming languages. |
It’s important to ensure your development environment is version-controlled to avoid conflicts between packages and libraries. Tools like Docker can help you manage consistent environments across different machines and teams.
Building Your First AI Model: From Data Collection to Preprocessing
Creating an AI model involves several key steps, starting from gathering the right data to preprocessing it effectively. The quality of the data you collect directly impacts the accuracy of your AI system. Without reliable and clean data, even the most advanced algorithms will perform poorly. Thus, the initial phase of data collection plays a critical role in building any AI model.
Once you have gathered your dataset, the next task is preprocessing. This step helps transform raw data into a structured format that is easier for algorithms to process. Preprocessing ensures that any inconsistencies, missing values, or irrelevant features are addressed, making the dataset suitable for model training.
Steps in Data Collection and Preprocessing
- Data Collection: Gather data from reliable sources. This could include datasets from open repositories or data scraped from websites, APIs, or sensors.
- Data Cleaning: Remove any duplicate, incomplete, or irrelevant data points to improve the quality of the dataset.
- Data Transformation: Convert data into a format suitable for model input (e.g., scaling numerical values or encoding categorical variables).
- Feature Engineering: Create new features or modify existing ones to better represent the underlying patterns in the data.
Effective data preprocessing is crucial for the performance of any AI model. Even minor issues in the data can lead to significant reductions in model accuracy.
Key Preprocessing Techniques
- Normalization: Adjust numerical data to a common scale, typically between 0 and 1.
- Handling Missing Values: Decide whether to impute missing values, remove rows/columns, or leave them as is, depending on the dataset.
- Encoding Categorical Variables: Use techniques like one-hot encoding or label encoding to represent non-numeric data.
Example: Preprocessing Table
Step | Action | Tools/Techniques |
---|---|---|
Data Cleaning | Remove duplicates and irrelevant columns | Pandas, NumPy |
Missing Data | Impute or drop missing values | Scikit-learn, Pandas |
Feature Engineering | Scale/transform features | Scikit-learn, Featuretools |
Designing Neural Networks: A Step-by-Step Guide
To successfully create a neural network, you need to focus on both the architecture and the data used for training. The design of the model can significantly impact its accuracy and efficiency, so understanding the necessary steps is essential. This process starts with data preparation, then moves on to selecting the appropriate network type and optimization techniques. Each step must be handled carefully to ensure that the model performs optimally.
This guide will provide a detailed overview of how to approach the creation of a neural network, from pre-processing data to fine-tuning the model. By following these steps, you will be able to build a robust network tailored to your specific task.
Step 1: Data Preparation
Before training a neural network, it is vital to preprocess the data properly. This process includes tasks such as:
- Scaling features to ensure uniformity across the input data.
- Cleaning the dataset by removing or imputing missing values.
- Splitting the dataset into training, validation, and test subsets.
Important: Data normalization helps improve model performance and ensures stable convergence during training.
Step 2: Network Architecture Selection
Choosing the correct architecture is crucial for a neural network's success. The network’s depth (number of layers) and the number of neurons per layer depend on the problem being solved. Here are some options:
- Fully Connected Networks (FCNs) are effective for general tasks.
- Convolutional Networks (CNNs) are best for image-related problems.
- Recurrent Networks (RNNs) excel in tasks involving sequential data, such as text or time series.
Note: Selecting the wrong architecture can lead to poor model performance and increased training times.
Step 3: Optimization Strategy
The choice of optimization algorithm is a key factor in training efficiency. Here are common methods:
Optimization Method | Description |
---|---|
Stochastic Gradient Descent (SGD) | Standard method, adjusting weights incrementally after each sample. |
Adam | Combines the benefits of momentum and adaptive learning rates, widely used for deep learning tasks. |
Tip: Adam is generally the preferred choice for deep learning applications due to its efficiency in handling large datasets.
Training Your AI Model with Real-World Data
When developing an AI model, one of the most important stages is the training phase, where the model learns from real-world data. The process involves feeding large amounts of relevant data into the system, allowing it to recognize patterns and make predictions or decisions. The quality and relevance of this data directly influence the performance of the AI, so it's crucial to select and preprocess the data carefully.
To ensure that your AI can generalize well and make accurate predictions in various situations, it's essential to gather data that closely represents the problem space it will operate in. This means not only using data from controlled environments but also considering noisy, incomplete, and unstructured data from real-world scenarios.
Key Steps in Training with Real-World Data
- Data Collection: Gather a diverse range of data from various sources that reflect the real-world conditions in which your AI will function.
- Data Cleaning: Handle missing or incorrect data, removing outliers and filling in gaps where necessary.
- Data Labeling: Label data accurately, ensuring that each data point is associated with the correct output, whether it's a classification or regression task.
- Data Augmentation: Apply transformations to the data (like rotation, scaling, etc.) to artificially increase the dataset's size and variety.
Real-world data is often messy, so careful preprocessing is vital to avoid introducing biases or inaccuracies that could impact the model's performance.
Data Split and Model Evaluation
To avoid overfitting and ensure the model's generalization capabilities, it's important to split the data into training, validation, and test sets. This approach allows the model to be trained on one subset of the data, fine-tuned using another, and evaluated on a separate, unseen portion.
- Training Set: Used to train the model, allowing it to learn patterns from the data.
- Validation Set: Used to adjust the model's parameters and avoid overfitting.
- Test Set: Evaluates the model's performance on new, unseen data to estimate how well it will perform in the real world.
Data Split | Purpose |
---|---|
Training Set | Used for training the model. |
Validation Set | Helps in tuning the model's hyperparameters. |
Test Set | Used to evaluate model performance after training. |
Optimizing Your AI Model for Better Performance
Optimizing the performance of an AI model is a critical step in ensuring that it meets the desired outcomes efficiently. This involves improving speed, accuracy, and resource usage. Optimization techniques can be applied at different stages of the model development cycle, including data processing, model architecture design, and post-training adjustments. Below are some essential practices to help fine-tune your AI model for maximum performance.
By focusing on reducing model complexity, selecting the right features, and implementing appropriate regularization, you can significantly enhance your AI model. Moreover, it's important to regularly evaluate performance through validation and testing, applying any necessary changes based on feedback and results.
Key Optimization Techniques
- Feature Engineering: Choosing the most relevant features improves model accuracy by eliminating irrelevant data.
- Regularization: Apply techniques like L1 and L2 regularization to avoid overfitting and enhance generalization.
- Hyperparameter Tuning: Experiment with different hyperparameter values to find the optimal configuration.
- Model Pruning: Reduce model size by removing unnecessary parameters or layers, leading to faster computation times.
Steps to Optimize Your AI Model
- Preprocessing Data: Clean and standardize data to remove noise and inconsistencies, which helps the model learn better.
- Choosing the Right Model Architecture: Select a model that fits the task at hand while balancing complexity and performance.
- Fine-tuning Hyperparameters: Use grid search or random search to experiment with different hyperparameters.
- Regular Evaluation: Continuously assess model performance on validation data to catch overfitting or underfitting early on.
Performance Metrics Comparison
Metric | Description | Impact on Optimization |
---|---|---|
Accuracy | Measures the percentage of correct predictions. | Improves when model is well-tuned and avoids bias. |
Precision | Focuses on the proportion of true positives among all predicted positives. | Crucial in models where false positives are costly. |
Recall | Measures the proportion of true positives among all actual positives. | Important when missing positives can lead to significant errors. |
Optimizing a model is an ongoing process, requiring regular updates based on new data and insights. Continuous refinement ensures long-term success and relevance.
Deploying and Testing Your AI System in a Live Environment
Once the AI system is developed and trained, the next critical step is its deployment and testing in a live environment. This phase ensures that the AI can operate effectively with real-world data and interact with external systems or users. It involves setting up infrastructure, integrating with existing workflows, and verifying that the model functions as intended. This process often reveals challenges that did not appear during the development or testing phases.
Deployment also requires monitoring and performance tracking to ensure the AI continues to perform well over time. Factors such as latency, system load, and failure recovery must be managed. Below are the key steps and considerations for successfully deploying and testing an AI system.
Deployment Process
- Prepare deployment infrastructure (cloud or on-premise servers)
- Integrate with existing applications or systems
- Ensure scalability and fault tolerance
- Set up monitoring and logging tools for real-time performance analysis
Testing in a Live Environment
Testing the AI system once deployed requires careful validation of its performance under real-world conditions. The following steps should be taken:
- Integration Testing: Ensure the AI works within the larger system ecosystem.
- Load Testing: Simulate high traffic or demand to assess system behavior under stress.
- Continuous Monitoring: Track performance metrics to catch issues early.
- Feedback Loops: Incorporate user feedback to improve AI responses and outputs.
Important: Real-time testing often uncovers unexpected challenges like data drift or edge cases that were not previously considered during development.
Key Performance Metrics
Metric | Purpose |
---|---|
Latency | Measures the time taken to process and respond to requests. |
Throughput | Quantifies the number of requests the system can handle within a given time frame. |
Accuracy | Evaluates the correctness of the AI’s predictions or actions. |