R Convert Vectors to Dataframe
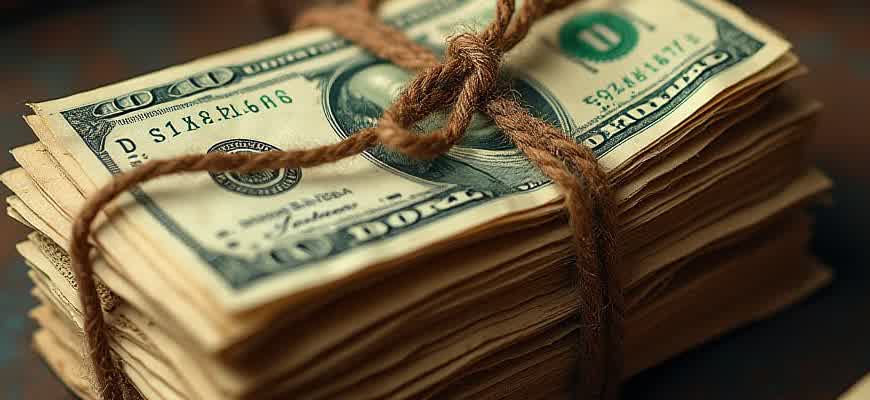
In R, vectors are one-dimensional arrays that hold elements of the same data type. However, there are situations when it is more practical to convert a vector into a data frame, which is a two-dimensional table structure capable of holding columns of different types of data. This allows better handling of complex datasets and is often a crucial step in data manipulation processes.
Here’s how you can perform this conversion:
- Use the data.frame() function to convert a vector into a data frame.
- Specify column names and other attributes to structure your data effectively.
- Ensure that the vector length aligns with the expected row count for consistency in the output.
Note: When working with larger datasets, converting vectors into data frames can greatly simplify the process of data analysis by allowing the use of row and column operations.
For example, you can convert a vector like this:
my_vector <- c(10, 20, 30, 40)
Into a data frame using the data.frame() function:
my_dataframe <- data.frame(Column1 = my_vector)
This will result in a table format, which can be easily manipulated or visualized:
Column1 |
---|
10 |
20 |
30 |
40 |
How to Convert Numeric Vectors into DataFrames in R
In R, working with vectors is a common practice, but at times, you might need to convert them into a more structured format like a DataFrame for better analysis and manipulation. This process is simple and can be achieved using several methods, depending on the structure and context of your data.
When converting numeric vectors to DataFrames, it is important to ensure that the resulting DataFrame represents the data in a way that can be efficiently processed. There are multiple ways to perform this conversion, and each method may offer advantages based on the data's complexity and format.
Basic Conversion Using DataFrame Function
The simplest way to convert a numeric vector into a DataFrame is by using the data.frame() function. Here's how to do it:
numeric_vector <- c(1, 2, 3, 4, 5)
df <- data.frame(numeric_vector)
This method directly converts the numeric vector into a DataFrame with a single column. By default, the column name will be taken from the name of the vector (if provided), or it will be labeled as numeric_vector.
Adding Column Names to DataFrame
If you need to name the column explicitly, you can do so by assigning names to the columns:
df <- data.frame(Number = numeric_vector)
This results in a DataFrame with the column labeled as Number.
Using Multiple Vectors to Create a DataFrame
If you want to combine multiple vectors into a DataFrame, you can do so by passing them as separate arguments within the data.frame() function:
vector1 <- c(1, 2, 3)
vector2 <- c(4, 5, 6)
df <- data.frame(Column1 = vector1, Column2 = vector2)
This will create a DataFrame with two columns: Column1 and Column2.
Important Considerations
When converting vectors into a DataFrame, it is crucial to check that all vectors are of the same length. If the vectors have differing lengths, R will recycle the shorter vectors, potentially leading to unexpected results.
Example Conversion with a Table
Here’s an example with multiple vectors:
Index | Height | Weight |
---|---|---|
1 | 170 | 65 |
2 | 180 | 75 |
3 | 160 | 55 |
height <- c(170, 180, 160)
weight <- c(65, 75, 55)
df <- data.frame(Index = 1:3, Height = height, Weight = weight)
In this case, the resulting DataFrame has three columns: Index, Height, and Weight.
Transforming Character Vectors into Data Frames in R: A Comprehensive Guide
In R, converting character vectors into data frames is a common operation, especially when working with raw data that is organized as lists or arrays. This transformation can help structure data for further analysis or visualization. Whether you're dealing with a single vector or multiple character vectors, R provides straightforward methods to convert them into data frames, making it easier to perform complex tasks such as subsetting, aggregating, or applying functions to columns.
R offers a variety of techniques to achieve this, depending on the specific structure of your input data. In this guide, we will explore step-by-step how to turn character vectors into data frames, ensuring the process is clear and manageable for both beginners and experienced users.
Steps to Convert a Character Vector into a Data Frame
Here are the key steps to follow for converting a character vector into a data frame:
- Create a character vector: You begin by defining your character vector that holds the data.
- Use the data.frame() function: This function allows you to convert the character vector into a structured data frame.
- Assign column names: Once the character vector is converted, you can assign meaningful column names to improve data readability.
For example, here is a simple illustration of this process:
# Create a character vector char_vector <- c("John", "Paul", "George", "Ringo") # Convert to data frame df <- data.frame(Name = char_vector) # View the result print(df)
Remember that the data frame created from a character vector will have one column by default, but you can add additional columns as needed.
Working with Multiple Character Vectors
When dealing with multiple character vectors, you can combine them into a single data frame. For example, you might have a vector for names and another for ages. By combining them, you can create a more complex data structure:
# Define multiple character vectors names <- c("John", "Paul", "George", "Ringo") ages <- c("40", "42", "38", "41") # Combine into a data frame df <- data.frame(Name = names, Age = ages) # View the result print(df)
Below is how the resulting data frame looks:
Name | Age |
---|---|
John | 40 |
Paul | 42 |
George | 38 |
Ringo | 41 |
Handling Missing Data When Converting Vectors to DataFrames
When working with vectors in R, it's common to encounter missing values, especially when converting vectors to data frames. In these situations, handling the missing data properly is crucial to ensure the integrity of your analysis. There are several methods available to address missing data, and the choice of method depends on the nature of the data and the analysis requirements.
Missing data can occur for various reasons, such as data entry errors, incomplete observations, or intentional omission. When converting vectors to data frames, R will often handle these missing values using the NA value, but how these missing values are treated can influence the results of subsequent data operations. Understanding how to manage missing data in this context is an essential skill for data manipulation in R.
Strategies for Handling Missing Data
- Omitting Missing Values: One common approach is to simply remove any rows or columns that contain missing data. While this is a straightforward solution, it can lead to loss of valuable information if the missing data is substantial.
- Imputing Missing Values: Another option is to fill in missing values with estimates. This can be done using mean imputation, median imputation, or more advanced techniques like multiple imputation, depending on the nature of the dataset.
- Leaving Missing Values as NA: Sometimes, it's preferable to leave missing values as NA and handle them separately in analysis. This is often the case in exploratory data analysis where the researcher needs to identify patterns in missingness.
Example of Missing Data Handling
Method | Pros | Cons |
---|---|---|
Omitting rows/columns | Simplicity, no imputation required | Loss of data, especially in small datasets |
Imputing values | Preserves data, makes analysis more complete | Imputation can introduce bias or inaccuracies |
Leaving NA | Can be useful for identifying patterns in missingness | May complicate analysis if not handled properly |
It’s important to test how different methods of handling missing data impact your analysis before making a final decision on the approach to use.
Using Custom Column Names During Data Frame Conversion
When transforming vectors into a data frame in R, one of the most common tasks is to assign specific names to the columns of the resulting data frame. By default, R will either use numeric column names or the names of the original vectors. However, you can easily assign custom names, which can enhance readability and make the data more intuitive to work with.
To assign custom column names, you can utilize the `colnames()` function or specify the names directly during the creation of the data frame. This is particularly useful when you want to maintain consistency in naming conventions or when the vector names are not descriptive enough for your analysis.
Using `colnames()` for Renaming Columns
One way to set custom column names is by first creating a data frame and then renaming the columns using the colnames()
function. Here's an example:
# Create a data frame
df <- data.frame(v1 = c(1, 2, 3), v2 = c(4, 5, 6))
# Rename columns
colnames(df) <- c("Column1", "Column2")
In this example, the columns of the data frame were renamed from v1
and v2
to Column1
and Column2
, making the data more meaningful.
Directly Assigning Column Names During Data Frame Creation
Alternatively, you can define custom column names while creating the data frame by passing a data.frame
object with named vectors:
# Create a data frame with custom column names
df <- data.frame(Column1 = c(1, 2, 3), Column2 = c(4, 5, 6))
This approach is quick and efficient, particularly when you already have predefined column names for your data.
Important: Custom column names should be meaningful and follow a consistent naming pattern to improve the clarity and manageability of your data frame.
Additional Tips for Custom Column Names
- Ensure column names are not too long or complex to avoid confusion.
- Use underscores (
_
) instead of spaces for better compatibility with functions that work with column names. - If you have many columns, consider using shorter, more concise names while maintaining clarity.
Example of Data Frame with Custom Columns
Column1 | Column2 |
---|---|
1 | 4 |
2 | 5 |
3 | 6 |
By defining clear and concise column names, the structure of the data frame becomes easier to interpret, especially when you are working with larger datasets or when you need to share the data with others. Using meaningful labels is a good practice for ensuring that the analysis remains transparent and reproducible.
Converting Multiple Vectors into a Single DataFrame
When working with R, it is common to have multiple vectors that represent different variables or measurements. The task of organizing these vectors into a structured format, such as a data frame, is essential for easier data manipulation and analysis. A data frame allows you to represent the data as a table with rows and columns, which is especially useful when dealing with datasets in statistical analysis or machine learning.
To combine multiple vectors into a single data frame, you can use the `data.frame()` function in R. Each vector can become a column in the data frame, and the elements within each vector will be arranged as rows under their respective column headers.
Method for Combining Vectors
The process of merging vectors into a data frame can be broken down into the following steps:
- Create individual vectors that you want to combine.
- Use the
data.frame()
function to combine them into one object. - Ensure that each vector represents one column, and all vectors are of the same length (or R will automatically recycle elements where possible).
Note: Ensure all vectors have the same number of elements, otherwise, R may recycle values to align them, which could lead to unintended results.
Here is an example of how this works:
Vector 1 | Vector 2 | Vector 3 |
---|---|---|
1 | A | TRUE |
2 | B | FALSE |
3 | C | TRUE |
The above table could be created in R with the following code:
vec1 <- c(1, 2, 3)
vec2 <- c("A", "B", "C")
vec3 <- c(TRUE, FALSE, TRUE)
df <- data.frame(vec1, vec2, vec3)
print(df)
By using this method, you can easily manage and manipulate multiple data columns simultaneously. The result will be a data frame with each vector represented as a column, making it much easier to perform analysis or visualization on the data.
Optimizing Data Types in DataFrame Conversion for Better Performance
When converting vectors to a DataFrame in R, one of the most crucial aspects to consider is the selection of appropriate data types. Choosing the right types for each column can significantly improve both memory usage and processing speed. Converting raw vectors into a structured DataFrame without paying attention to the underlying data types often leads to inefficiencies. These inefficiencies arise from unnecessary overhead associated with incorrectly assigned data types, which can degrade performance, especially with large datasets.
Efficiently optimizing the data types requires an understanding of the operations that will be performed on the DataFrame. If columns are incorrectly converted to broader types like factor or character when more specific types (e.g., integer, numeric) would suffice, this can lead to increased memory consumption and slower processing. By optimizing these types, you can reduce the overall size of your DataFrame, making data manipulation faster and more memory-efficient.
Key Strategies for Optimizing Data Types
- Use the most appropriate type: For numerical data, prefer numeric or integer over character or factor types.
- Convert factors to character only when necessary: Factors are useful for categorical data, but they can add unnecessary overhead if used incorrectly.
- Explicitly define column types: When creating a DataFrame, specify the column types using colClasses to avoid R’s automatic, and sometimes suboptimal, type assignment.
Practical Example of Data Type Optimization
Consider a situation where you are creating a DataFrame from vectors representing IDs, names, and scores. The ID column should be an integer, the name column should be a character, and the scores should be numeric. Optimizing the data types would involve defining these types explicitly.
Column | Suggested Data Type |
---|---|
ID | Integer |
Name | Character |
Score | Numeric |
Tip: Always use the most specific data type possible to avoid unnecessary memory consumption and ensure fast computations. For instance, if you know your ID values will always be positive integers, avoid using factors or numeric types.
Additional Considerations
- Memory profiling: Use the pryr::mem_used() function to check the memory usage before and after type optimization.
- Data cleaning: Clean your data before converting it into a DataFrame, as this can ensure the types are consistent and avoid potential errors during conversion.
Managing Row Names When Converting Vectors to DataFrames
When converting vectors into a DataFrame in R, it is crucial to handle row names correctly to maintain data integrity. In many cases, row names are automatically generated, but they may not always represent meaningful identifiers or may need adjustments. Knowing how to control and customize row names is key to ensuring that the final DataFrame structure is both accurate and useful.
Understanding how row names are treated during the conversion process can save time and prevent errors. The default behavior is that the row names are assigned sequentially, starting from 1, unless explicitly defined by the user. However, if a vector contains specific names, they may be automatically assigned as row names, or the user may want to redefine them after conversion.
Common Methods to Control Row Names
- Default Row Names: If no specific row names are provided, R will assign integer values starting from 1.
- Manual Assignment: Users can manually assign row names during the creation of the DataFrame using the rownames() function.
- Vector with Named Elements: If the vector has named elements, these names are used as row names when the vector is converted to a DataFrame.
Practical Example
Here's a simple example to demonstrate how row names can be managed during conversion:
# Example 1: Converting a vector without row names my_vector <- c(10, 20, 30) my_dataframe <- data.frame(my_vector) # Example 2: Assigning custom row names rownames(my_dataframe) <- c("A", "B", "C")
Potential Pitfalls
While working with row names, some challenges may arise:
- Duplicated Row Names: If the vector contains duplicate names, it may lead to unexpected behavior or errors when performing certain operations.
- Inconsistent Row Names: If row names are manually assigned after the DataFrame is created, it is important to ensure the number of names matches the number of rows.
Remember that row names should be unique and correspond to the number of rows in the DataFrame to avoid inconsistencies during analysis.
Summary of Key Points
Method | Effect |
---|---|
Default Assignment | Assigns integer values starting from 1 |
Manual Assignment | Allows users to specify custom row names |
Named Vector | Uses the vector’s names as row names |
Common Mistakes When Transforming Vectors into DataFrames and How to Avoid Them
Converting vectors into DataFrames is a common operation in R, but there are several pitfalls that can lead to unexpected results or errors. These issues are often related to mismatched data types, incorrect assumptions about the structure of the data, and the misunderstanding of how the vector elements are mapped into the DataFrame's rows and columns. Awareness of these common errors can help you prevent problems and ensure smooth data transformation.
Understanding the underlying data structure and ensuring that vectors are properly formatted before conversion is key to avoiding issues. Below are some of the most common mistakes when converting vectors to DataFrames, along with strategies to mitigate them.
1. Mismatched Vector Lengths
One of the most common errors is when vectors of different lengths are combined into a DataFrame. This results in incomplete rows or additional missing values, which can lead to incorrect analysis or visualizations.
Tip: Always ensure that all vectors have the same length before attempting to combine them into a DataFrame.
- Check if all vectors are of equal length.
- If they are not, you may need to adjust your data or use NA values to fill the missing spots.
2. Inconsistent Data Types
When combining vectors with different data types (e.g., numeric and character), R may attempt to coerce the entire DataFrame to a common type, potentially leading to unintended results. For instance, a DataFrame might incorrectly treat numeric data as characters.
Tip: Always check the data type of your vectors using the str()
function and convert them to appropriate types if necessary before creating the DataFrame.
- Use the
as.
function to explicitly convert the data to the desired type. - For numerical data, convert strings to numbers using
as.numeric()
if required.
3. Improper Column Naming
Another common issue arises when column names are either missing or incorrectly assigned. Without proper column names, it can be challenging to interpret the data or use functions that rely on column names.
Tip: Explicitly define column names when converting vectors, or use the colnames()
function to assign meaningful names.
Vector | Column Name |
---|---|
1, 2, 3 | Age |
John, Jane, Mike | Name |