C Convert Argv to Int
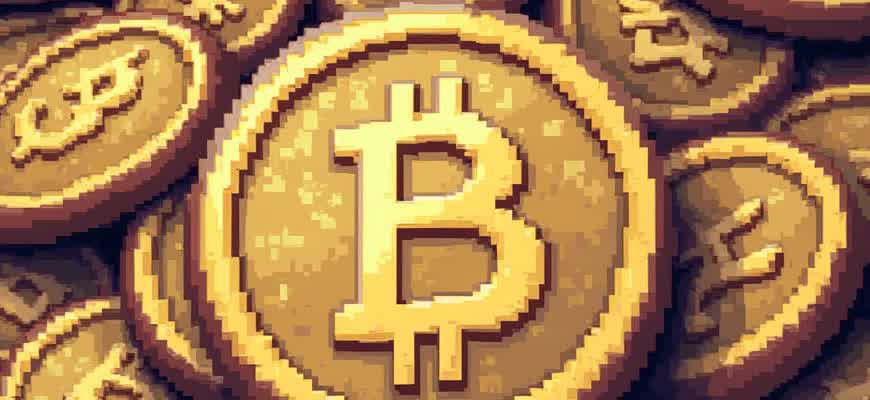
In C programming, when running a program through the command line, the arguments passed to it are stored as strings in the argv array. To process numerical values from these arguments, it is essential to convert them into integer types. This conversion can be achieved using several built-in functions, depending on the expected input format.
There are two primary methods for converting the values in argv to integers:
- Using atoi() function
- Using strtol() function
Note: It’s crucial to handle invalid input properly, as functions like atoi() do not provide error handling, whereas strtol() offers more control.
The table below summarizes the key differences between the two functions:
Function | Returns | Error Handling |
---|---|---|
atoi() | Integer | None |
strtol() | Long Integer | Provides error detection via errno and return value checks |
How to Convert Command-Line Arguments to Integers in C
When working with C programs, converting command-line arguments to integers is a common requirement. Command-line arguments are passed to the `main` function as an array of strings, where each string represents an argument passed to the program. Often, you need to convert these strings to integer values for processing. This conversion can be done using standard library functions like `atoi()` or `strtol()`. It is essential to ensure that the conversion is done correctly, as improper conversion could lead to errors or unexpected behavior in the program.
To handle the conversion properly, you must consider various scenarios, such as handling invalid input or exceeding the range of integer values. This guide will walk you through the steps involved in converting command-line arguments to integers and explain how to handle possible issues along the way.
Steps for Converting Command-Line Arguments to Integers
- Pass the command-line arguments to the `main` function. The arguments are stored as strings in the `argv` array.
- Use a function like `atoi()` or `strtol()` to convert the string to an integer.
- Check if the conversion was successful. For `atoi()`, if the input is not a valid number, it returns `0`, so additional checks might be required to ensure correct conversion.
Common Functions for Conversion
- atoi(): Converts a string to an integer, but doesn't handle errors well.
- strtol(): More robust than `atoi()`. It provides error checking and can handle long integers.
Note: While `atoi()` is simple to use, it does not give any indication of an invalid input. For better error handling, use `strtol()` instead.
Example Conversion Using strtol
Here’s an example of how to safely convert a command-line argument to an integer using `strtol()`:
#include#include int main(int argc, char *argv[]) { if (argc != 2) { printf("Usage: %s \n", argv[0]); return 1; } char *endptr; long num = strtol(argv[1], &endptr, 10); if (*endptr != '\0') { printf("Invalid input: %s is not a valid integer.\n", argv[1]); return 1; } printf("Converted number: %ld\n", num); return 0; }
Comparison of Conversion Functions
Function | Error Handling | Range |
---|---|---|
atoi() | None | Limited to int range |
strtol() | Reports errors (invalid input, out-of-range) | Long range, better for large numbers |
Why Convert Command-Line Arguments to Integers in C?
When writing programs that take user input via command-line arguments, it’s often necessary to handle numeric data. Command-line arguments are passed as strings, which means that any numerical input needs to be converted into integers for correct processing. Without converting these arguments, mathematical operations or logical checks on numeric values would not be possible.
Furthermore, working with raw strings can lead to errors, especially when performing calculations or comparisons. By converting the input arguments into integers, you ensure that the program operates on the actual data type needed for computation, improving both functionality and stability.
Key Reasons for Conversion
- Data Integrity: Ensures the program handles numbers correctly, preventing errors like concatenating digits instead of performing arithmetic.
- Input Validation: Converting helps verify that the input is indeed a valid number and avoids issues related to string-based manipulation.
- Efficient Calculations: Once converted to integers, numerical data can be used in mathematical operations, enabling the program to function as intended.
Steps for Conversion
- Use atoi or strtol: These C functions are typically used to convert a string to an integer.
- Check for Errors: Always validate if the conversion is successful, as invalid input can lead to undefined behavior.
- Handle Edge Cases: Take care of edge cases, such as out-of-range values or non-numeric characters, to prevent program crashes.
Example Conversion Function
Input String | Converted Integer |
---|---|
"123" | 123 |
"-456" | -456 |
"abc" | 0 |
Note: Always ensure that the input string is sanitized and contains a valid number before conversion to avoid unexpected results.
Setting Up the C Environment for Argument Conversion
Before starting with argument conversion in C, it’s essential to have the appropriate environment in place. This involves ensuring that your development tools and libraries are correctly configured to handle command-line arguments. The standard C library provides all necessary functions to work with input from the command line, specifically `argc` and `argv`. Proper setup of your development environment ensures smooth compilation and execution of your program.
The next step is to configure your IDE or text editor, ensuring that you can easily compile and run C programs. This might involve setting up environment variables or installing necessary compilers such as GCC. Once the environment is prepared, you can begin working with command-line arguments in C.
Steps for Configuring Your Development Environment
- Install a C compiler such as GCC or Clang.
- Choose a text editor or IDE that supports C programming (e.g., Visual Studio Code, CLion, or Vim).
- Ensure that your terminal or command prompt is correctly configured to compile C files.
- Check if the necessary libraries (like stdlib.h and stdio.h) are available for use in your projects.
Basic Compilation Process
- Write your C program in a text editor or IDE.
- Save the file with a `.c` extension (e.g., `main.c`).
- Open the terminal and navigate to the directory containing the C file.
- Compile the code using a command like:
gcc -o program main.c
. - Run the compiled program with arguments:
./program 5 10 15
.
Important Note
Be sure to test your environment setup thoroughly. Errors in environment configuration can result in issues with compiling or running programs.
Common Errors and Troubleshooting
Error | Possible Cause | Solution |
---|---|---|
“gcc: command not found” | GCC is not installed or the PATH is incorrect. | Install GCC and configure the PATH environment variable. |
“undefined reference to `main’” | The program lacks a main function or is misconfigured. | Ensure the program contains a valid int main(int argc, char *argv[]) function. |
Understanding the Syntax for `argv` in C
When working with command-line arguments in C, the `argv` array is essential for accessing inputs provided by the user when executing a program. These arguments are passed to the program during its execution and are stored as strings within the `argv` array, which is part of the main function's parameters.
The `argv` array allows the program to handle user input dynamically. Each element in the array corresponds to a separate argument, with the first element typically being the name of the program itself. Understanding its syntax and proper usage is crucial for parsing and processing command-line arguments effectively in C programs.
How `argv` Works
The syntax for using `argv` in C is straightforward, but requires proper indexing to access the arguments correctly:
- Argument Count (`argc`): The first parameter, typically named `argc`, stores the number of arguments passed to the program, including the program's name.
- Argument Values (`argv`): The second parameter, `argv`, is an array of strings, where each string represents an individual argument. `argv[0]` is always the name of the program, and subsequent indices (`argv[1]`, `argv[2]`, etc.) hold the user-provided arguments.
Example of `argv` in Use
The following code snippet demonstrates how to access command-line arguments in C:
#includeint main(int argc, char *argv[]) { for(int i = 0; i < argc; i++) { printf("Argument %d: %s\n", i, argv[i]); } return 0; }
In this example, the program will print out all the arguments passed to it, including the name of the program itself.
Note that when converting `argv` values from strings to integers, functions like
atoi()
orstrtol()
are commonly used.
Structure of the `argv` Array
Index | Value |
---|---|
argv[0] | Program name |
argv[1] | First argument passed |
argv[2] | Second argument passed |
argv[argc-1] | Last argument passed |
Using `atoi()` and `strtol()` for Argument Parsing
When working with command-line arguments in C, it is often necessary to convert string inputs into integer values. Two commonly used functions for this purpose are `atoi()` and `strtol()`. While both functions provide a way to parse string arguments into integers, they have key differences that can influence their usage depending on the situation.
The `atoi()` function is a simple solution for converting a string to an integer, but it has several limitations. It returns 0 when the conversion fails, making it difficult to detect errors. On the other hand, `strtol()` offers more flexibility and error-handling capabilities, allowing for better control over the parsing process.
Key Differences Between `atoi()` and `strtol()`
- Return value:
- atoi(): Returns the integer value or 0 if conversion fails.
- strtol(): Returns the converted long integer, and sets a pointer to the first invalid character encountered, making error detection easier.
- Error handling:
- atoi(): Does not provide error reporting for invalid input.
- strtol(): Provides error handling by setting the `errno` variable and allowing the caller to detect failures through its second argument.
- Handling large numbers:
- atoi(): May fail or return unexpected results when parsing very large or very small integers.
- strtol(): Handles large numbers better, with overflow or underflow conditions properly flagged.
When to Use Each Function
- Use `atoi()`:
- When you need a quick, simple conversion and are certain the input is valid.
- When you are handling basic cases with no need for complex error detection.
- Use `strtol()`:
- When you need more robust error handling, especially when the input might be malformed.
- When working with larger numbers or when you need to detect overflow or underflow conditions.
Example Usage of `atoi()` and `strtol()`
Function | Example Code |
---|---|
atoi() | int num = atoi(argv[1]); |
strtol() | char *endptr; long num = strtol(argv[1], &endptr, 10); |
Tip: Use `strtol()` if you require better error checking and support for a wider range of numeric inputs.
Handling Invalid Input and Conversion Errors
When dealing with command-line arguments in C, it's essential to ensure that the input is both valid and correctly formatted. This becomes particularly important when converting string arguments into integer values. Invalid input can result in errors that may crash the program or lead to unexpected behavior. Therefore, proper error handling mechanisms must be in place to detect and manage such situations effectively.
One of the most common errors when converting strings to integers is the inability to parse the input correctly. If the input string contains non-numeric characters or is empty, it may lead to conversion failures. Handling these scenarios prevents runtime errors and ensures the program behaves as expected under different conditions.
Common Errors and Their Solutions
- Non-Numeric Input: If the input string contains characters that cannot be converted to an integer, functions like
strtol
oratoi
will fail. A reliable way to handle this is to check the result of the conversion and validate if it matches the expected range of integers. - Empty Strings: Empty arguments can occur when the user omits values. It's important to check whether the string is empty before attempting conversion.
- Overflow or Underflow: Integer overflow or underflow occurs when the number exceeds the limits of the integer data type. This can be detected by checking if the result of the conversion is within the range defined for integers.
Example: Error Handling in Input Conversion
- Check if the argument count is correct before processing.
- Use functions like
strtol
for safe conversion and check for errors usingerrno
. - Validate the converted integer to ensure it's within the acceptable range.
- Display error messages if invalid input is detected, and exit gracefully.
Tip: Always usestrtol
overatoi
for better error handling.strtol
provides more control and returns additional error information that can be useful for debugging.
Error Handling Table
Error Type | Cause | Solution |
---|---|---|
Non-Numeric Input | Input contains characters other than digits | Use strtol and check for conversion errors using errno |
Empty String | No value provided for conversion | Check for empty input before processing |
Overflow/Underflow | The value is too large or small to be represented as an integer | Validate the result against INT_MAX and INT_MIN |
Converting Multiple Command Line Arguments to Integers
When working with command line inputs, it is common to deal with multiple arguments passed to a program. In C programming, these arguments are typically received as strings. Converting these strings to integers is necessary for mathematical operations and further processing. Handling multiple arguments efficiently requires attention to detail and the proper use of conversion functions.
To convert multiple arguments at once, we can utilize loops and built-in functions such as atoi() or strtol(). A typical approach involves iterating through the arguments, applying the conversion function to each, and storing the results. This process ensures that each argument is individually processed and accurately transformed into an integer.
Iterative Conversion Method
One efficient way to handle multiple arguments is by using a loop. Below is an example of how we can convert each command-line argument into an integer:
- Initialize a loop to go through each argument.
- Apply the conversion function to each argument.
- Store the result in an integer array or process it immediately.
Note: Always check for invalid inputs, such as non-numeric characters, to avoid runtime errors during conversion.
Example: Converting Arguments to Integers
Here’s a simple example to demonstrate how to convert multiple arguments passed through the command line:
#include
#include
int main(int argc, char *argv[]) {
for (int i = 1; i < argc; i++) {
int num = atoi(argv[i]);
printf("Argument %d converted to integer: %d\n", i, num);
}
return 0;
}
Alternative Approach: Using strtol()
Another method of conversion is using strtol(), which provides better error handling. This function allows you to specify a base and checks for invalid characters more effectively:
#include
#include
int main(int argc, char *argv[]) {
char *endptr;
for (int i = 1; i < argc; i++) {
long int num = strtol(argv[i], &endptr, 10);
if (*endptr != '\0') {
printf("Invalid number: %s\n", argv[i]);
} else {
printf("Argument %d converted to integer: %ld\n", i, num);
}
}
return 0;
}
Important Considerations
While performing conversions, keep the following in mind:
- Use proper error handling to account for invalid or malformed inputs.
- Ensure that each argument is processed correctly by checking the return value of conversion functions.
- Consider edge cases such as large numbers or non-numeric input.
Summary of Methods
Method | Advantages | Disadvantages |
---|---|---|
atoi() | Simple and easy to use. | Does not handle errors effectively. |
strtol() | Better error handling and flexibility. | More complex to implement. |
Optimizing the Argument Conversion Process
In C programming, converting command-line arguments to integers is a routine but essential task. The conversion process, however, can become inefficient if not handled correctly. By focusing on performance, we can reduce the computational overhead and handle large inputs effectively. Several techniques can help streamline this process, ensuring that we minimize errors and improve the overall execution speed of the program.
One of the key strategies to enhance the conversion process is using optimized functions and error-checking mechanisms. Rather than relying on standard functions like atoi()
, which does not handle errors well, utilizing strtol()
provides better error handling and allows more control over the conversion. Additionally, minimizing the number of unnecessary function calls can significantly boost performance in cases where many arguments need to be processed.
Techniques for Efficient Conversion
- Use
strtol()
orstrtoll()
instead ofatoi()
for safer conversions. - Check for overflow or underflow using
errno
or range checks after conversion. - Limit the use of loops that iterate over arguments, as these can add overhead in larger programs.
- Perform pre-validation of arguments before conversion to catch errors early.
Important Considerations
Note: Always ensure that arguments are valid integers before attempting conversion. Invalid input can cause unexpected behavior, especially when performing mathematical operations afterward.
Example Conversion Efficiency
Function | Error Handling | Performance |
---|---|---|
atoi() |
Poor | Fast but risky due to lack of error checking |
strtol() |
Good | Slower but more reliable and error-resistant |
strtoll() |
Good | Suitable for handling long integers with error checking |
Conclusion
- Choose the right conversion function based on error handling and the expected input range.
- Pre-validate inputs to avoid unnecessary overhead during conversion.
- Optimize loops by processing arguments efficiently to minimize execution time.
Real-World Use Cases for Argument Conversion in C Programs
In C programming, converting command-line arguments to integers is often required for tasks where input values need to be processed or validated before being used in calculations or program logic. One common scenario is when a program needs to process numerical data passed by the user, ensuring that the input is appropriately converted from string representations to actual integer values. This is particularly relevant for applications like calculators, configuration settings, or even complex data processing tools that require user input for initialization or operation.
Additionally, argument conversion in C programs is important for handling real-world situations where command-line inputs dictate the behavior of the application. These inputs can range from simple values like file paths or flags to more complex numerical data, such as port numbers or memory sizes. Proper conversion ensures that the program can function as intended, without errors or incorrect behavior due to invalid or improperly formatted inputs.
Key Use Cases
- Command-Line Calculators: A user may provide a mathematical operation and numbers as arguments, such as "add 5 10". The program converts these arguments to integers and performs the requested calculation.
- Configuration Parameters: Programs often accept numerical parameters, like timeouts or memory limits, which need to be converted from string inputs to integers for use in system configuration.
- Networking Applications: Server applications that require port numbers or IP addresses as arguments need to convert strings to integers to set up connections or bind to specific ports.
Example: Argument Conversion in Networking
A common scenario in networking applications involves converting a user-provided port number (given as a string) into an integer that is then used by the program to establish network connections.
- Input: "server 8080"
- Action: Convert the string "8080" to the integer 8080
- Output: The program uses the integer 8080 as the port number to establish the server connection
Table: Argument Conversion in Action
Argument | Conversion Method | Use Case |
---|---|---|
"100" | atoi() or strtol() | Configuring a timeout value in a program |
"8080" | atoi() or strtol() | Setting up a server to listen on a specific port |
"256MB" | strtol() with appropriate validation | Allocating memory based on user input |